
Python
Python Crash Course
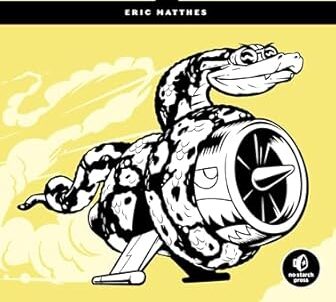
Project URL: https://github.com/Alex-Scott-Tech
Working through the first half of the book "Python Crash Course" by Eric Mathes. In this project I am learning how to:
1. Introduction to Python
- Overview of Python and its applications.
- Setting up Python and a development environment.
- Introduction to coding concepts and the importance of programming.
2. Basic Concepts
- Data Types: Strings, integers, floats, and booleans.
- Variables: Creating and using variables.
- Lists: Creating, accessing, and modifying lists; list methods.
- Tuples and Sets: Understanding tuples and sets and their differences from lists.
3. Control Structures
- Conditionals: Using
if
,elif
, andelse
statements to control program flow. - Loops:
for
andwhile
loops, usingbreak
andcontinue
. - List Comprehensions: Simplifying list creation and manipulation.
4. Functions
- Defining and calling functions.
- Passing arguments and returning values.
- Understanding scope and lifetime of variables.
5. Dictionaries
- Creating and using dictionaries.
- Accessing and modifying dictionary values.
- Dictionary methods and common use cases.
6. User Input and While Loops
- Collecting user input with
input()
. - Using
while
loops for repeated actions. - Validating input and handling errors.
7. Working with Files
- Reading from and writing to files.
- Understanding file methods and file handling.
- Working with JSON data.
8. Classes and Objects
- Introduction to Object-Oriented Programming (OOP).
- Defining classes and creating instances.
- Attributes and methods, inheritance, and working with multiple classes.